|
Computer-aided systems, whose behavior is not hardcoded and who can therefore later adapt their behavior to an environment, are called adaptive systems. These are therefore capable of learning, in a way. Industrial robots are “trained” by specialists in a "teach mode", for instance which arm movements are to be carried out. In most cases, the operator uses an input system similar to a remote control. The robot is successively moved to the desired positions and the respective state is stored.
In "execution mode" the robot runs through the stored states independently (and with a higher speed).
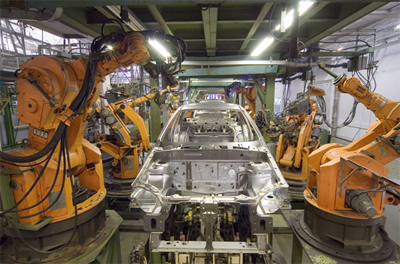
As before, your canal robot knows the constant length of the canal elements, but its turning movements are controlled by a human. However, the robot is now able to learn, so it can memorize the path and independently run through it any number of times.
It is often useful to imagine that in every moment, a robot is in a particular state. The states are typically labeled with meaningful words and stored as a string. You assume the following states: the robot is stopped, moving forward, turning left, or right, and you call them: STOPPED, FORWARD, LEFT, RIGHT. [more...
It would be better to use an enumerated type (enum) for the states. In the version of Python on the EV3 but they are missing]
Instead of constantly querying the keys or buttons, here you use a more elegant event programming model with registered callback functions, which are, independently of the currently running program, always called automatically when an event occurs.
The main program, in an endless loop, is engaged in performing the corresponding actions in each state. The state change takes place in the callback onButtonHit().
|
from simrobot import *
#from nxtrobot import *
#from ev3robot import *
RobotContext.useObstacle("sprites/bg.gif", 250, 250)
RobotContext.setStartPosition(310, 470)
RobotContext.showStatusBar(30)
def onButtonHit(buttonID):
global state
if buttonID == BrickButton.ID_LEFT:
state = "LEFT"
elif buttonID == BrickButton.ID_RIGHT:
state = "RIGHT"
elif buttonID == BrickButton.ID_ENTER:
state = "RUN"
moveTime = 3200
turnTime = 545
memory = []
robot = LegoRobot(buttonHit = onButtonHit)
gear = Gear()
robot.addPart(gear)
state = "FORWARD"
while not robot.isEscapeHit():
if state == "FORWARD":
robot.drawString("Moving forward", 0, 3)
gear.forward(moveTime)
state = "STOPPED"
robot.drawString("Teach me!", 0, 3)
elif state == "LEFT":
memory.append(0)
robot.drawString("Saved: LEFT-TURN", 0, 3)
gear.left(turnTime)
state = "FORWARD"
elif state == "RIGHT":
memory.append(1)
robot.drawString("Saved: RIGHT-TURN", 0, 3)
gear.right(turnTime)
state = "FORWARD"
elif state == "RUN":
robot.drawString("Executing memory", 0, 1)
robot.drawString(str(memory), 0, 2)
robot.reset()
robot.drawString("Moving forward", 0, 3)
gear.forward(moveTime)
for k in memory:
if k == 0:
robot.drawString("Turning left", 0, 3)
gear.left(turnTime)
else:
robot.drawString("Turning right", 0, 3)
gear.right(turnTime)
robot.drawString("Moving forward", 0, 3)
gear.forward(moveTime)
gear.stop()
robot.drawString("All done", 0, 3)
state = "STOPPED"
robot.exit()
|
Highlight program code
(Ctrl+C to copy, Ctrl+V to paste) |
|